Delay Function In Dev C
To use delay function in your program you should include the 'dos.h' header file which is not a part of standard C library. Delay in C program If you don't wish to use delay function then you can use loops to produce delay in a C program. Insert, wherever you need your program to make a delay: sleep (1000); Change the '1000' to the number of milliseconds you want to wait (for example, if you want to make a 2 second delay, replace it with '2000'. Tip: On some systems the value might refer to seconds, instead of milliseconds. So sometimes 1000 isn't one second, but, in fact. In this post, we will see how to give a time delay in C code. Basic idea is to get current clock and add the required delay to that clock, till current clock is less then required clock run an empty loop. Here is implementation with a delay function.
Delay in C: delay function is used to suspend execution of a program for a particular time.
Declaration: void delay(unsigned int);
Here unsigned int is the number of milliseconds (remember 1 second = 1000 milliseconds). To use delay function in your program you should include the 'dos.h' header file which is not a part of standard C library.
Delay in C program
If you don't wish to use delay function then you can use loops to produce delay in a C program.
#include<stdio.h>int main()
{
int c, d;
for(c =1; c <=32767; c++)
for(d =1; d <=32767; d++)
{}
return0;
}
We have not written any statement in the loop body. You may write some statements that doesn't affect logic of the program.
C programming code for delay
#include<stdio.h>#include<stdlib.h>
main()
{
printf('This C program will exit in 10 seconds.n');
delay(10000);
return0;
}
This C program exits in ten seconds, after the printf function is executed the program waits for 10000 milliseconds or 10 seconds and then it terminates.
While Linux provides a multitude of system calls and functions to providing various timing and sleeping functions, sometimes it can be quite confusing, especially if you are new to Linux, so the ones presented here mimic those available on the Arduino platform, making porting code and writing new code somewhat easier.
Note: Even if you are not using any of the input/output functions you still need to call one of the wiringPi setup functions – just use wiringPiSetupSys() if you don’t need root access in your program and remember to #include <wiringPi.h>
- unsigned int millis (void)
This returns a number representing the number of milliseconds since your program called one of the wiringPiSetup functions. It returns an unsigned 32-bit number which wraps after 49 days.
- unsigned int micros (void)
This returns a number representing the number of microseconds since your program called one of the wiringPiSetup functions. It returns an unsigned 32-bit number which wraps after approximately 71 minutes.
- void delay (unsigned int howLong)
This causes program execution to pause for at least howLongmilliseconds. Due to the multi-tasking nature of Linux it could be longer. Note that the maximum delay is an unsigned 32-bit integer or approximately 49 days.
Delay Function In Dev Circuit
- void delayMicroseconds (unsigned int howLong)
Delay Function In Dev C++
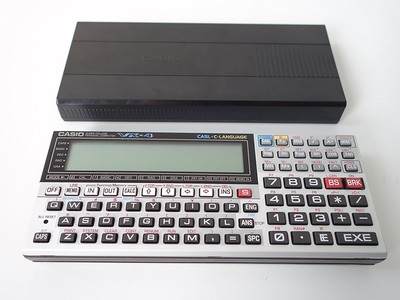
This causes program execution to pause for at least howLongmicroseconds. Due to the multi-tasking nature of Linux it could be longer. Note that the maximum delay is an unsigned 32-bit integer microseconds or approximately 71 minutes.
Delay Function In Dev Command
Delays under 100 microseconds are timed using a hard-coded loop continually polling the system time, Delays over 100 microseconds are done using the system nanosleep() function – You may need to consider the implications of very short delays on the overall performance of the system, especially if using threads.